.NET 9 Preview 1
已经发布,在.NET 9 中,新增了一些功能,包括JSON序列化、LINQ、Cryptography、SignalR和字典(Dictionary)调试等。本文介绍.NET 9中JSON的一些变化。
JsonSerializerOptions 的 Web 默认值
在System.Text.Json
中,.NET 9为序列化JSON提供了新的选项,并且增加了一个新的单例,这使得使用Web默认设置进行序列化变得更加容易。在 .NET 9 及更高版本中,可使用 JsonSerializerOptions.Web
单一实例通过 ASP.NET Core 用于 Web 应用的默认选项进行序列化。
代码如下:
public class Forecast{
public DateTime? Date { get; init; }
public int TemperatureC { get; set; }
public string? Summary { get; set; }}Forecast forecast = new(){
Date = DateTime.Now,
TemperatureC = 40,
Summary = "Hot"};JsonSerializerOptions options = new(JsonSerializerDefaults.Web){
WriteIndented = true};
string webJson = JsonSerializer.Serialize(
new { SomeValue = 42 },
JsonSerializerOptions.Web // Defaults to camelCase naming policy.
);Console.WriteLine(webJson);// {"someValue":42}
.NET 9 中的JsonSerializerOptions
包含一些新属性,可用于自定义写入 JSON 的缩进字符和缩进大小。例如:
var options = new JsonSerializerOptions{
WriteIndented = true,
IndentCharacter = '\t',
IndentSize = 2,};string json = JsonSerializer.Serialize(
new { Value = 1 },
options );Console.WriteLine(json);
Linq引入了两个新的方法CountBy
和AggregateBy
。CountBy
用于快速计算每个键出现频率的方法。AggregateBy
用于将数据分组,并对每个组执行聚合操作(例如求平均值、计数等)。
string sourceText = """
Lorem ipsum dolor sit amet, consectetur adipiscing elit.
Sed non risus. Suspendisse lectus tortor, dignissim sit amet,
adipiscing nec, ultricies sed, dolor. Cras elementum ultrices amet diam.""";KeyValuePair<string, int> mostFrequentWord = sourceText .Split(new char[] { ' ', '.', ',' }, StringSplitOptions.RemoveEmptyEntries)
.Select(word => word.ToLowerInvariant())
.CountBy(word => word)
.MaxBy(pair => pair.Value);Console.WriteLine(mostFrequentWord.Key); // amet
上面代码会计算出给定文本中出现次数最多的单词。其中
sourceText
是一段需要统计的文本。
.Split(new char[] { ' ', '.', ',' }, StringSplitOptions.RemoveEmptyEntries)
,将文本按照空格、句号和逗号进行分割,同时去除空字符串。
.Select(word => word.ToLowerInvariant())
将每个单词转换为小写字母。
使用.CountBy(word => word)
对字符串集合进行分组,每个组包含相同单词的单词列表,返回一个字典数据结构 KeyValuePair<string, int>。键 (key) 是单词,值 (value) 是出现次数。
最后使用MaxBy(pair => pair.Value)
对字典进行排序。最终结果是amet
出现的最多。
(string id, int score)[] data =
[
("0", 42),
("1", 5),
("2", 4),
("1", 10),
("0", 25),
];var aggregatedData =
data.AggregateBy(
keySelector: entry => entry.id,
seed: 0,
(totalScore, curr) => totalScore + curr.score );foreach (var item in aggregatedData){
Console.WriteLine(item);}//(0, 67)//(1, 15)//(2, 4)
上面代码比较简单,将定义好的数组,通过AggregateBy
方法对数组data
进行聚合操作。得到最终结果
//(0, 67)//(1, 15)//(2, 4)
Cryptography
增加了新的静态哈希方法,例如:
byte[] hash = CryptographicOperations.HashData(hashAlgorithmName, data);
这样就可以省钱new来new去的操作。
Cryptography
还增加了一个KMAC算法
,简单示例如下:
byte[] key = GetKmacKey();byte[] input = GetInputToMac();byte[] mac = Kmac128.HashData(key, input, outputLength: 32);
KMAC
使用Keccak
哈希函数,而HMAC
使用SHA
哈希函数。 Keccak
被认为是更安全的哈希函数,因为它具有更高的抗碰撞性。因此KMAC
被认为比HMAC
更安全,因为它使用了两轮哈希函数,并采用了密钥填充技术。
目前互联网上KMAC
的讨论比较少,因为它是一个较新的算法,所以兼容性等方面还差一些。如果是对安全性较高的场景,如金融交易、身份认证等方面,使用KMAC
是个不错的选择。
SignalR
中心方法现在可接受基类(而不是派生类)来实现多态方案。
字典和其他键值集合的调试显示具有改进的布局。 键显示在调试程序的键列中,而不是与值连接在一起。 下图显示了调试程序中字典的旧显示和新显示。
.之前:
以后:
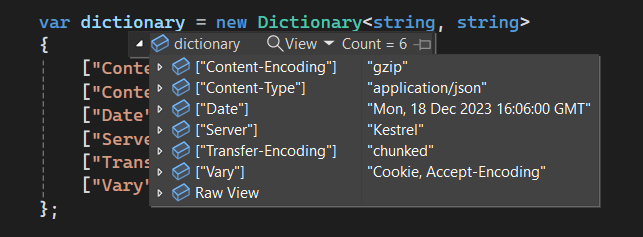
好啦,目前.NET 9 Preview 1
的新增功能就说这么多了,其他零碎的新功能就不多说了,之后的文章会再进行跟进。
最后说一下,.NET 9跟.NET 7一样,是标准期限支持
。所以呢,还是等长期支持的.NET 10吧!